Sending Emails with Django using SendGrid in 3 easy steps
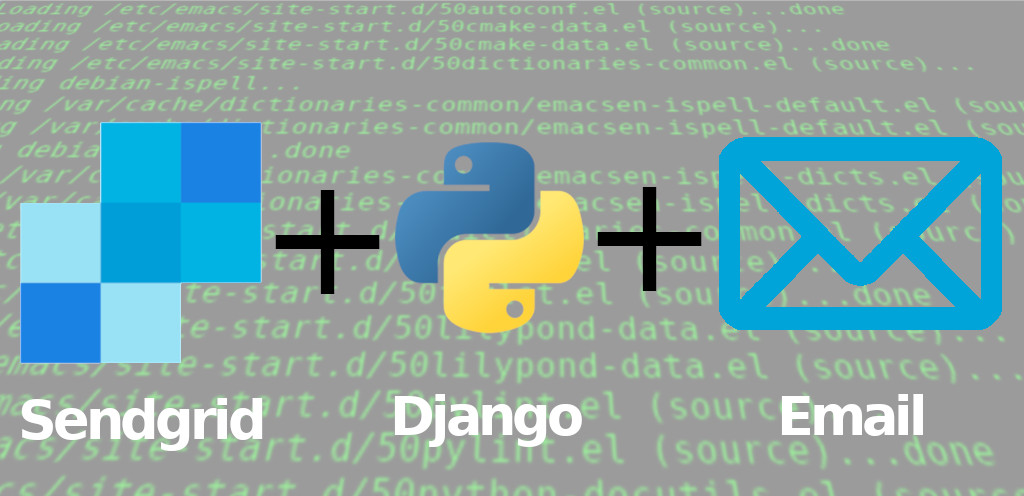
Overview
Step by step guide to provide your Django app with Email capabilities.
Typically used for account activation and notifications.
This guide uses one of the most popular solutions, SendGrid, which provides a considerably free amount of emails for free each month (100 per month at the time of writing this article).
Using Sendgrid with Django
Email service can be added to Django following two strategies:
- Using SendGrid’s Web API or
- Using SMTP as the transport mechanism
In this tutorial we are going to use the first method, with a Web API. 1
To use the Web API we will rely on the the django-sendgrid-v5 package,
This package implements an email backend for Django that relies on *SendGrid*'s REST API for message delivery.
Steps
Log in to Sendgrid at https://app.sendgrid.com/login
1. Get API keys
And Create an API key for the app at https://app.sendgrid.com/settings/api_keys
We will create an API key just for sending emails, so in Name
enter my_app_send_mails
.
Then choose Restricted Access, enable Mail Send and hit the Create button.
A new API Key will be generated, copy and set the SENDGRID_API_KEY
environment variable.
Here you can use dot-env or
django-dotenv approaches,
so /.env
would look like:
SENDGRID_API_KEY=XXXXXXXXXXXXXXXXXXXXXXXX
2. Use package
Using pip we install django-sendgrid-v5: pip install django-sendgrid-v5
And then tell Django to use it.
3. Configure Keys in Django
in app/settings.py
:
import os
EMAIL_BACKEND = "sendgrid_backend.SendgridBackend"
SENDGRID_API_KEY = os.environ.get("SENDGRID_API_KEY")
Additional
There are also optional settings to deliver emails in debug mode or to send them to standard output:
# Toggle sandbox mode (when running in DEBUG mode)
SENDGRID_SANDBOX_MODE_IN_DEBUG=True
# echo to stdout or any other file-like object that is passed to the backend via the stream kwarg.
SENDGRID_ECHO_TO_STDOUT=True
Testing it works
To test that everything works fine we will send an email.
Out of sandbox
First turn off SendGrid’s sandbox mode SENDGRID_SANDBOX_MODE_IN_DEBUG=True
so we can send and receive an email from console.
Send an email
Then test sending the email with django.core.mail.send_mail
like:
from django.core.mail import send_mail
send_mail(
'Subject here',
'Here is the message.',
'from@example.com',
['to@example.com'],
fail_silently=False,
)
Putting it all together:
$ python3 manage.py shell >>> from django.core.mail import send_mail >>> send_mail('testing', 'my message', 'hello@mydomain.com', ['personal@example.com'], fail_silently=False) .virtualenvs/xxx/lib/python3.6/site-packages/sendgrid_backend/mail.py:53: UserWarning: Sendgrid email backend is in sandbox mode! Emails will not be delivered. warnings.warn("Sendgrid email backend is in sandbox mode! Emails will not be delivered.") 1 >>> from django.conf import settings >>> settings.SENDGRID_SANDBOX_MODE_IN_DEBUG=False >>> send_mail('testing', 'my message', 'hello@mydomain.com', ['personal@example.com'], fail_silently=False) 1
And you should get an email at peronsal@example.com.
Sender Authentication
We can also make some improvements, like Domain Authentication at https://app.sendgrid.com/settings/sender_auth to improve deliverability by proving to inbox providers that you own the domain you’re sending from.
Send emails from own domain
Also, instead of sending emails from accounts like …@sendgrid.net, it would be better to have them with our domain …@mydomain.com. In SendGrid this is called Link Branding and can be configured at: https://app.sendgrid.com/settings/sender_auth/link/create
References
- https://docs.djangoproject.com/en/2.1/topics/email/
send_mail
: https://docs.djangoproject.com/en/2.1/topics/email/#send-mail- https://sendgrid.com/docs/for-developers/sending-email/django
If you want to use SMTP sending email over SMTP with Django check Sendgrid smtp tutorial with Django email guide. ↩︎
- August 1, 2023
- How to create a reusable Django app and distribute it with PIP or publish to pypi.orgJune 29, 2021
- How To Serve Multiple Django Applications with uWSGI and Nginx in Ubuntu 20.04October 26, 2020
- How to add favicon to Django in 4 stepsSeptember 3, 2020
- Categories in Django with BreadcrumbsAugust 30, 2020
- How To Migrate From SQLite To PostgreSQL In Django In 3 stepsAugust 28, 2020
- Practical guide to internationalize a Django app in 5 steps.August 24, 2020
- Disable new users singup when using Django's allauth packageSeptember 3, 2019
- How to add ads.txt to Django as requested by Google AdsenseAugust 30, 2019
- Have multiple submit buttons for the same Django formJuly 2, 2019
- Better Testing with Page Object Design in DjangoMay 1, 2019
- Generating slugs automatically in Django without packages - Two easy and solid approachesFebruary 14, 2019
- How to set up Django tests to use a free PostgreSQL database in HerokuFebruary 13, 2019
- Dynamically adding forms to a Django FormSet with an add button using jQueryFebruary 6, 2019
- Use of Django's static templatetag in css file to set a background imageFebruary 1, 2019
- Activate Django's manage.py commands completion in Bash in 2 stepsJanuary 29, 2019
- Sending Emails with Django using SendGrid in 3 easy steps
- Adding Users to Your Django Project With A Custom User ModelSeptember 21, 2018
- Setting Up A Factory For One To Many Relationships In FactoryboyApril 17, 2018
- Generate UML class diagrams from django modelsMarch 24, 2018
- Set Up Ubuntu To Serve A Django Website Step By StepJuly 3, 2017
- Django Project Directory StructureJuly 16, 2016
- How to Have Different Django Settings for Development and Production, and environment isolationJune 10, 2016
- Django OverviewJune 2, 2016
Django Forms
- Adding a Cancel button in Django class-based views, editing views and formsJuly 15, 2019
- Using Django Model Primary Key in Custom Forms THE RIGHT WAYJuly 13, 2019
- Django formset handling with class based views, custom errors and validationJuly 4, 2019
- How To Use Bootstrap 4 In Django FormsMay 25, 2018
- Understanding Django FormsApril 30, 2018
- How To Create A Form In DjangoJuly 29, 2016
Articles
Subcategories
Except as otherwise noted, the content of this page is licensed under CC BY-NC-ND 4.0 . Terms and Policy.
Powered by SimpleIT Hugo Theme
·