How to add ads.txt to Django as requested by Google Adsense
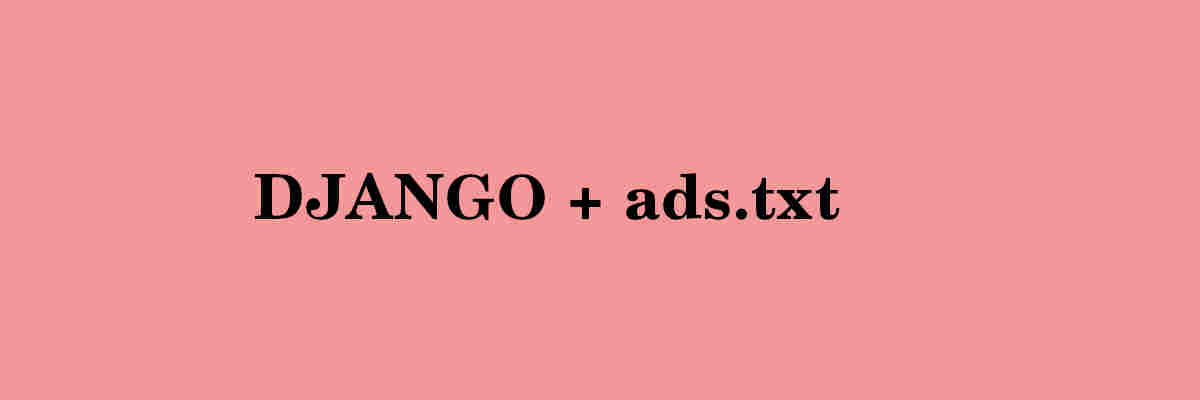
Three methods to add ads.txt
Overview
As Google Adsense adopted the Authorized Digital Sellers, or ads.txt standard, each Django website needs an ads.txt
file at
root.
Ads.txt is a simple, flexible, and secure method for publishers and distributors to declare who is authorized to sell their inventory, improving transparency for programmatic buyers.
Here we explore three alternatives to serve this file correctly.
- With a redirect or
- Return a single line response
- Serve directly from
nginx
The first method uses the file you download from Google, and has the
flexibility of adding more sellers and maintaining a proper ads.txt
file. While the second approach is a bit hacky and it just serves a
single line of ads.txt
, which is also the most common scenario.
This will solve the infamous Adsense warning message: Earnings at risk - One or more of your sites does not have an ads.txt file
Download ads.txt
Go to your Adsense’s homepage, then sites/my-sites and download the ads.txt file.
It will consist of a single line like this:
google.com, pub-0000000000000000, DIRECT, f08c47fec0942fa0
where pub-0000000000000000
is your own publisher
ID.
1. Redirect method
1.2 Static folder
Put the ads.txt
file in your static folder, typically it would be
/static
, you can check the value of the settings.STATIC_ROOT
variable1.
1.3 Redirect
Your static files will be served using the path specified at
STATIC_URL,
for example, in your app/settings.py
you would have something like:
STATIC_URL = "/static/"
This is the URL to use when referring to static files located in
STATIC_ROOT
(after running collectstatic
). The problem with this
is that ads.txt
needs to be located at the root of your website
https://example.com/ads.txt
and not at
https://example.com/static/ads.txt
.
To address this we do a redirect
RedirectView.as_view(url=staticfiles_storage.url("ads.txt"))
each
time there is a request to /ads.txt
, in app/urls.py
:
"""equilang URL Configuration
"""
from django.contrib.staticfiles.storage import staticfiles_storage
from django.urls import include, path
from django.views.generic.base import RedirectView
from django.conf import settings
from django.conf.urls.static import static
urlpatterns = [
//...
path(
"ads.txt",
RedirectView.as_view(url=staticfiles_storage.url("ads.txt")),
),
] + static(settings.MEDIA_URL, document_root=settings.MEDIA_ROOT)
The above import django.contrib.staticfiles.storage.url
, is used to
select the right storage backend, based in
settings.STATICFILES_STORAGE
variable’s value.
It also uses the Generic Class Based View django.views.generic.base.RedirectView to redirect to the specified URL.
Note
Google supports redirects when looking for the ads.txt
file.
Google supports a single HTTP redirect to a destination outside the original root domain (for example, example1.com/ads.txt re-directs to example2.com/ads.txt). See the IAB update.
Multiple redirects are also supported, as long as each redirect location remains within the original root domain. For example:
example.com/ads.txt redirecting to example.com/page/ads.txt
2. Response method
2.1 Ads.txt view
We add a view that returns a single line response, In myapp/views.py
:
from django.http import HttpResponse
from django.views import View
class AdsView(View):
"""Replace pub-0000000000000000 with your own publisher ID"""
line = "google.com, pub-0000000000000000, DIRECT, f08c47fec0942fa0"
def get(self, request, *args, **kwargs):
return HttpResponse(line)
2.2 URL
Then we need to return the above response when there is request to
/ads.txt
, so in myapp/urls.py
:
from django.urls import path
from myapp.views import AdsView
urlpatterns = [
path('ads.txt', AdsView.as_view()),
]
3. Nginx method
3.1 Static folder
Put the ads.txt
file in your static folder, typically it would be /static
.
3.2 Configure nginx
Using the
location
directive, which sets configuration depending on a request URI, we
define an
alias
directive to serve the ads.txt
file.
In your nginx website virtual host configuration:
location /ads.txt {
alias /staticfiles/ads.txt;
}
Conclusion
The above tutorial steps may differ a bit from your specific static settings but the general idea is pretty simple.
Now every request to https://example.com/ads.txt
will be served by
https://example.com/static/ads.txt
.
I prefer the first method, using the original file, as you can have the flexibility of maintaining that file outside the app source code, adding or removing sellers as you need.
Happy Adsensing! 🤑
References
- https://iabtechlab.com/ads-txt-about/
- https://support.google.com/adsense/answer/7532444?hl=en
- https://docs.djangoproject.com/en/2.0/ref/settings/#std:setting-STATIC_URL
- https://docs.djangoproject.com/en/2.2/ref/settings/#staticfiles-storage
- https://docs.djangoproject.com/en/2.2/howto/static-files/
- August 1, 2023
- How to create a reusable Django app and distribute it with PIP or publish to pypi.orgJune 29, 2021
- How To Serve Multiple Django Applications with uWSGI and Nginx in Ubuntu 20.04October 26, 2020
- How to add favicon to Django in 4 stepsSeptember 3, 2020
- Categories in Django with BreadcrumbsAugust 30, 2020
- How To Migrate From SQLite To PostgreSQL In Django In 3 stepsAugust 28, 2020
- Practical guide to internationalize a Django app in 5 steps.August 24, 2020
- Disable new users singup when using Django's allauth packageSeptember 3, 2019
- How to add ads.txt to Django as requested by Google Adsense
- Have multiple submit buttons for the same Django formJuly 2, 2019
- Better Testing with Page Object Design in DjangoMay 1, 2019
- Generating slugs automatically in Django without packages - Two easy and solid approachesFebruary 14, 2019
- How to set up Django tests to use a free PostgreSQL database in HerokuFebruary 13, 2019
- Dynamically adding forms to a Django FormSet with an add button using jQueryFebruary 6, 2019
- Use of Django's static templatetag in css file to set a background imageFebruary 1, 2019
- Activate Django's manage.py commands completion in Bash in 2 stepsJanuary 29, 2019
- Sending Emails with Django using SendGrid in 3 easy stepsJanuary 9, 2019
- Adding Users to Your Django Project With A Custom User ModelSeptember 21, 2018
- Setting Up A Factory For One To Many Relationships In FactoryboyApril 17, 2018
- Generate UML class diagrams from django modelsMarch 24, 2018
- Set Up Ubuntu To Serve A Django Website Step By StepJuly 3, 2017
- Django Project Directory StructureJuly 16, 2016
- How to Have Different Django Settings for Development and Production, and environment isolationJune 10, 2016
- Django OverviewJune 2, 2016
Django Forms
- Adding a Cancel button in Django class-based views, editing views and formsJuly 15, 2019
- Using Django Model Primary Key in Custom Forms THE RIGHT WAYJuly 13, 2019
- Django formset handling with class based views, custom errors and validationJuly 4, 2019
- How To Use Bootstrap 4 In Django FormsMay 25, 2018
- Understanding Django FormsApril 30, 2018
- How To Create A Form In DjangoJuly 29, 2016
Articles
Subcategories
Except as otherwise noted, the content of this page is licensed under CC BY-NC-ND 4.0 . Terms and Policy.
Powered by SimpleIT Hugo Theme
·