Solve Selenium WebDriverException executable needs to be in PATH error message
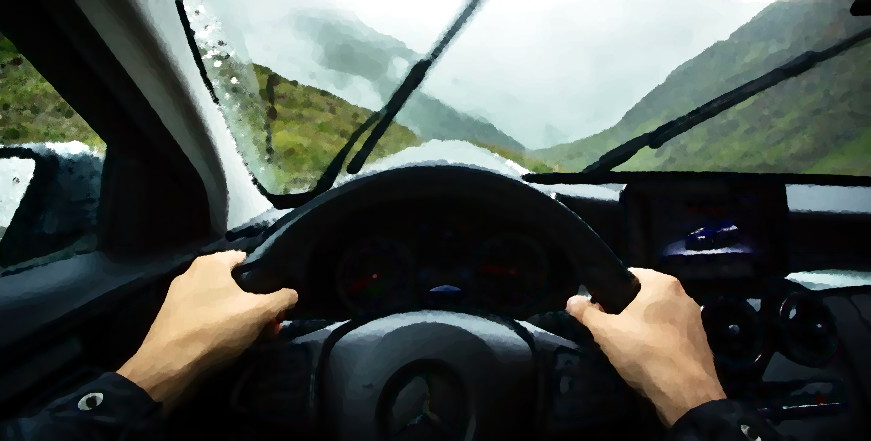
Works for Chrome (chromedriver), Firefox (geckodriver) and the rest of possible drivers available in Selenium
Overview
Guide to use Selenium with the appropriate web driver.
In Selenium we have to choose a specific “browser” to use in our code, for example if we want to use Chrome:
from selenium.webdriver.chrome.webdriver import WebDriver
browser = WebDriver()
The above code expects that we already have the right drivers to execute the browser in our code, if not it will display an error message.
In this case we solve Selenium’s error message about not being able
to find the desired set of capabilities used in our code:
selenium.common.exceptions.SessionNotCreatedException: Message: Unable to find a matching set of capabilities
.
Or when it can’t find the right webdriver it will show some of the following errors:
- Using Chrome:
selenium.common.exceptions.WebDriverException: Message: 'chromedriver' executable needs to be in PATH. Please see https://sites.google.com/a/chromium.org/chromedriver/home
- Using WebKitWebDriver:
selenium.common.exceptions.WebDriverException: Message: 'WebKitWebDriver' executable needs to be in PATH.
- Using Firefox:
selenium.common.exceptions.GeckoDriverException: Message: GeckoWebDriver' executable needs to be in PATH.
Solution
The generic steps to solve this are:
- download the desired browser driver executable
- make Selenium aware of the above driver location
1. First alternative: Automatic
The easiest way is to use webdriver-manager1 which is a library “to automatically manage drivers for different browsers”.
1.1 Install
Install with the command: pip install webdriver_manager
$ pip install webdriver_manager
Collecting webdriver-manager
Downloading webdriver_manager-2.4.0-py2.py3-none-any.whl (13 kB)
Requirement already satisfied: requests in /usr/local/lib/python3.6/dist-packages (from webdriver-manager) (2.22.0)
Collecting crayons
Using cached crayons-0.3.0-py2.py3-none-any.whl (4.6 kB)
Collecting configparser
Using cached configparser-5.0.0-py3-none-any.whl (22 kB)
Requirement already satisfied: urllib3!=1.25.0,!=1.25.1,1.26,=1.21.1 in /usr/local/lib/python3.6/dist-packages (from requests->webdriver-manager) (1.25.6)
Requirement already satisfied: chardet3.1.0,=3.0.2 in ~/.local/lib/python3.6/site-packages (from requests->webdriver-manager) (3.0.4)
Requirement already satisfied: certifi=2017.4.17 in /usr/local/lib/python3.6/dist-packages (from requests->webdriver-manager) (2019.9.11)
Requirement already satisfied: idna2.9,>=2.5 in ~/.local/lib/python3.6/site-packages (from requests->webdriver-manager) (2.8)
Requirement already satisfied: colorama in /usr/local/lib/python3.6/dist-packages (from crayons->webdriver-manager) (0.4.1)
Installing collected packages: crayons, configparser, webdriver-manager
Successfully installed configparser-5.0.0 crayons-0.3.0 webdriver-manager-2.4.0
1.2 Use
In your code use the right manager for Selenium’s webdriver:
pip install webdriver-manager
from selenium import webdriver
And then Choose the right browser
Chrome
For Chrome:
from webdriver_manager.chrome import ChromeDriverManager
browser = webdriver.Chrome(ChromeDriverManager().install())
Firefox
For Firefox:
from webdriver_manager.firefox import GeckoDriverManager
browser = webdriver.Firefox(executable_path=GeckoDriverManager().install())
Internet Explorer
For IE:
from webdriver_manager.microsoft import IEDriverManager
browser = webdriver.Ie(IEDriverManager().install())
Edge
from webdriver_manager.microsoft import EdgeChromiumDriverManager
browser = webdriver.Edge(EdgeChromiumDriverManager().install())
2. Second alternative: Manual
If you don’t want to use an external package you can do it manually with one of these options.
First, download the right driver consulting your desired browser’s driver at: https://selenium-python.readthedocs.io/api.html
Then, one of these two alternatives:
- add driver location to system’s path
PATH=/location/of/browserdriver:$PATH
- specify the location via
Webdriver
’sexecutable_path
parameterndriver = webdriver.Chrome(executable_path='/location/of/firefoxdriver)
References
- Solve Selenium WebDriverException executable needs to be in PATH error message
- Pip upgrade all packages at once with a one-liner command January 31, 2019
- Test Files Creating a Temporal Directory in Python UnittestsSeptember 2, 2018
- How to Translate a Python Project With Gettext the Easy WayAugust 29, 2018
Behave Testing
Django webframework
- August 1, 2023
- How to create a reusable Django app and distribute it with PIP or publish to pypi.orgJune 29, 2021
- How To Serve Multiple Django Applications with uWSGI and Nginx in Ubuntu 20.04October 26, 2020
- How to add favicon to Django in 4 stepsSeptember 3, 2020
- Categories in Django with BreadcrumbsAugust 30, 2020
- How To Migrate From SQLite To PostgreSQL In Django In 3 stepsAugust 28, 2020
- Practical guide to internationalize a Django app in 5 steps.August 24, 2020
- Disable new users singup when using Django's allauth packageSeptember 3, 2019
- How to add ads.txt to Django as requested by Google AdsenseAugust 30, 2019
- Have multiple submit buttons for the same Django formJuly 2, 2019
- Better Testing with Page Object Design in DjangoMay 1, 2019
- Generating slugs automatically in Django without packages - Two easy and solid approachesFebruary 14, 2019
- How to set up Django tests to use a free PostgreSQL database in HerokuFebruary 13, 2019
- Dynamically adding forms to a Django FormSet with an add button using jQueryFebruary 6, 2019
- Use of Django's static templatetag in css file to set a background imageFebruary 1, 2019
- Activate Django's manage.py commands completion in Bash in 2 stepsJanuary 29, 2019
- Sending Emails with Django using SendGrid in 3 easy stepsJanuary 9, 2019
- Adding Users to Your Django Project With A Custom User ModelSeptember 21, 2018
- Setting Up A Factory For One To Many Relationships In FactoryboyApril 17, 2018
- Generate UML class diagrams from django modelsMarch 24, 2018
- Set Up Ubuntu To Serve A Django Website Step By StepJuly 3, 2017
- Django Project Directory StructureJuly 16, 2016
- How to Have Different Django Settings for Development and Production, and environment isolationJune 10, 2016
- Django OverviewJune 2, 2016
Django Forms
- Adding a Cancel button in Django class-based views, editing views and formsJuly 15, 2019
- Using Django Model Primary Key in Custom Forms THE RIGHT WAYJuly 13, 2019
- Django formset handling with class based views, custom errors and validationJuly 4, 2019
- How To Use Bootstrap 4 In Django FormsMay 25, 2018
- Understanding Django FormsApril 30, 2018
- How To Create A Form In DjangoJuly 29, 2016
Flask web microframework
- Understanding Flask's context conceptJanuary 26, 2017
- Avoid Using Flask Instance Folder When Deploying To HerokuJanuary 24, 2017
- Managing Environment Configuration Variables In Flask With DotenvJanuary 24, 2017
- Organize A Flask Project To Handle Production And Development Environments EffectivelyJanuary 11, 2017
- An Overview Of Flask Main Concepts And How It WorksDecember 31, 2016
Python Language
- Python Tools To Write Better CodeNovember 7, 2017
- Python Language Main Concepts And SummaryJune 11, 2017
- Python notesMay 30, 2016
Python Language Concepts
- Understanding How Python Packages Modules And Imports WorkJanuary 2, 2017
- Python Language Basic ConceptsJune 14, 2016
Python Environment
- Understanding Python 3 virtual environments different approachesJanuary 15, 2019
- Python Projects Isolation Using Virtual EnvironmentsJune 10, 2016
Articles
Subcategories
Except as otherwise noted, the content of this page is licensed under CC BY-NC-ND 4.0 . Terms and Policy.
Powered by SimpleIT Hugo Theme
·